What You Might Not Know about Chrome DevTools
Part One? Chrome's DevTools is a magical and mysterious beast. We can conquer this beast together! Josh Bavari recently gave a Lightning Talk on JS debugging. You should check it out. He is a great presenter.
Console API #
Let's start off with the Console API. Josh mentions a few in his presentation but there are so much more! The console object contains a lot more than just console.log()
.
Count #
There's a good chance you have done something like console.log(i + " Times")
in a loop to count the number of iterations. Instead you could do console.count('My Loop Name')
and you will get an output like this:
My Loop Name: 1
My Loop Name: 2
My Other Loop: 1
My Loop Name: 3
My Other Loop: 2
The number increments each time the function with that label runs. Each label has a counter so go wild creating as many as you need.
Formatting #
You can also format and even style some* of the console statements. By format I mean a printf
kind of API. If you are familiar with printf
then you are just curious what the format specifiers are.
Format Specifiers: #
%s
- String.%d
or%i
- Integer.%f
- Floating point number.%o
- DOM Element.%O
- Object.%c
- The secret to styling console statements.
How about an example?
console.log('My Array Name: %s Contents: %O Length: %i', 'stuff', stuff, stuff.length);
This example will output the following in the console: If you are wondering why I added
stuff.length
to my output string because it is in the %O
, I needed an integer for my example. That is all.
Now let's talk about styling a little. I have never used it in a practical application. The styling uses CSS. All you have to do is add the %c
format specifier to the output string and add some CSS as the value. An example would illustrate this better. I am just going to extend the previous example and make it pretty
console.log(
'%cMy Array Name: %s Contents: %O Length: %i',
'color: #663399; font-size: 2em;',
'stuff',
stuff,
stuff.length
);
This example will output the following in the console: Look at that huge purple output. Hard to miss, huh?
Group #
You can also group console statements with methods like console.group()
and console.groupCollapsed()
. These methods will group any log statements between it and a console.groupEnd()
method. This can be helpful if you are logging out a lot of data. A lot of times I will wrap the contents of a function I am debugging to make things easier to read. It is important to note that this does not work if there is an asynchronous call inside the group. It will appear outside of the group.
Time #
Need to time something? Look no further than console.time(label)
, console.timeline(label)
, or console.timeStamp([label])
. Time and timeline have similar functionality; timeStamp is a little different. Time and timeline both have *End()
methods. Time will output the time elapsed between the two method calls. Timeline starts the DevTools Timeline for the amount of time between start and end methods.
TimeStamp will just give you a marker at the current time on the Timeline with the specified label. This allows you to see what happening on the Timeline at that timestamp.
If you need a high precision time for profiling look into the Performance API. Also see this article about JavaScript Precision Timing.
Console Tab #
Right clicking on the Console section of DevTools to reveal two options. The first option is "Log XMLHttpRequests" and will output the HTTP method and the URL of the request. I can not express how much this has helped me while developing the SPA app that had heavy API integration. I have been working on the past few months.
The second option is "Preserve Log upon Navigation". This option allows you to debug issues where a redirect occurs. Recently, I used these on an issue was one of my API calls was throwing a 401 status code and causing a redirection to the login page. It was simple to track down which call using both of these options.
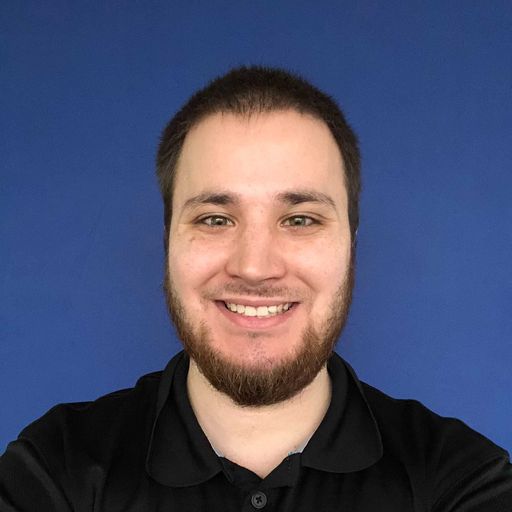