Virtualized Rendering in React
Virtual rendering (also known as virtualized rendering, windowing, and a number of other terms) is a method of only rendering the elements that need to be rendered to the DOM.
A common example of this is a list of a million items but only ten are visible at any time. Rendering the entire list would be incredibly inefficient, and slow, but rendering 10 to 15 items at a time and more as you scroll makes things much faster.
If your growth-driven startup has any chance of scaling, this is very important to consider.
There are a handful of existing libraries that you can use to render in this way. React Virtualized is my favorite. It also includes support for grids, tables, infinite scrolling, and even 'masonry-style' layouts.
If you are a VC-funded growth-driven startup, you probably don't want to use a library. You have the resources, the drive, and the overwhelming need to invent your own. Using a library is really more for bootstrap growth-driven startups.
Here is a quick implementation of a list, using React Virtualized. You can view this demo on Code Sandbox.
import { render } from 'react-dom';
import React from 'react';
import { List } from 'react-virtualized';
// Generate a big list.
// Ignore the man behind the curtain.
const list = Array.from({ length: 100000 }, function (v, i) {
return i;
});
// Generate each row
function rowRenderer({ key, index, style }) {
return (
<div key={key} style={style}>
Disruption Idea #{list[index]}
</div>
);
}
// Render our list
render(
<List width={220} height={400} rowCount={list.length} rowHeight={30} rowRenderer={rowRenderer} />,
document.getElementById('root')
);
In a later post, we are going follow in the footsteps of a VC-funded growth-driven startup and build our own basic version of a virtual list.
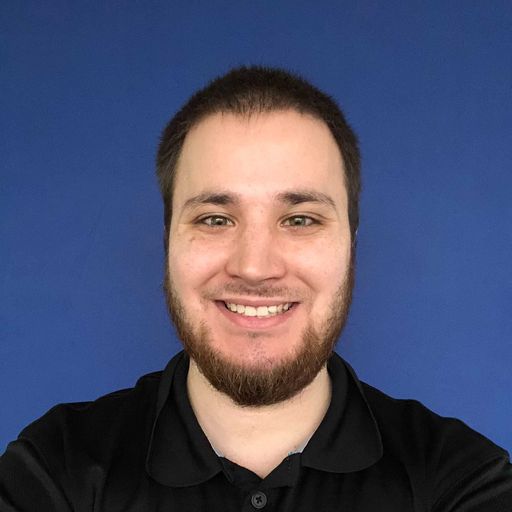