Deep Objects in Lodash
A quick introduction to two Lodash methods I found recently, _.get
and _.set
, to help work with deeply nested objects.
Let's say I have this object and I want to get the name.
var a = {
b: [
{
c: {
name: 'Arya',
},
},
],
};
Normally, we would be able to do a.b[0].c.name
but what if one of the values turned out to be null? This is common when we are waiting for data from an HTTP request or another source. We usually solve this by constructing this big mess that checks for falsy values at each step.
var name = a && a.b && a.b[0] && a.b[0].c && a.b[0].c.name;
// => 'Arya'
Let's clean this up using Lodash's get
method.
var name = _.get(a, 'b[0].c.name');
// => 'Arya'
We can also provide a default value as the third argument.
var name = _.get(a, 'b[0].c.name', 'A girl has no name');
// => 'Arya'
var name = _.get(a, 'b[1].c.name', 'A girl has no name');
// => 'A girl has no name'
I really like how much this cleans up the code.
We can also generate the object using _.set
.
var a = {};
_.set(a, 'b[0].c.name', 'Arya');
// => {b: [{c: {name: 'Arya'}}]}
It is important to note that _.set
mutates the passed object. To solve this, I would do something like this.
var a = {};
var new_a = _.set(Object.assign({}, a), 'b[0].c.name', 'Arya');
// => {b: [{c: {name: 'Arya'}}]}
To read more about these methods, check out the documentation for get and set.
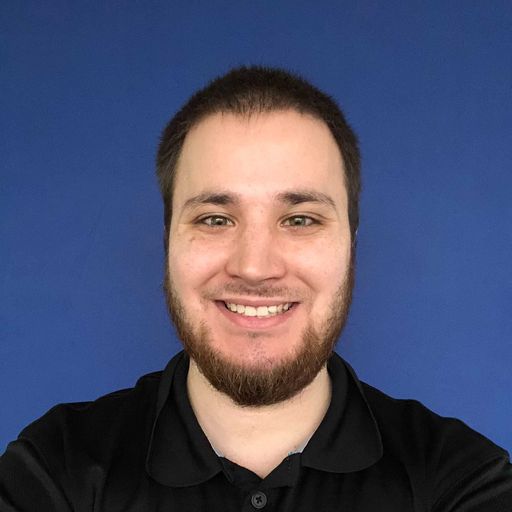