Use Fetch
How do you make an HTTP request in JavaScript? Do you pull in jQuery? Write your own XMLHttpRequest? There's a better way.
Enter fetch.
function toJSON(response) {
return response.json();
}
window
.fetch('http://catfacts-api.appspot.com/api/facts')
.then(toJSON)
.then(function (response) {
console.log(response);
// => {facts: ["Cat families usually play best in even numbers. Cats and kittens should be acquired in pairs whenever possible."], success: "true"}
});
This code will make a GET request to the catfacts API, parse the response as JSON, and log out the response to the console.
The bad news is support isn't super amazing with some browsers (as of November 2016). The good news is there are a handful of polyfills. whatwg-fetch by Github is a great one and it is a lot smaller than jQuery (and probably smaller than your XMLHttpRequest wrapper if it is feature-complete).
You can also use this in Node (and we do at WDT) using isomorphic-fetch.
The things I love about fetch
is that it has the API of a lot of the ajax wrappers out there.
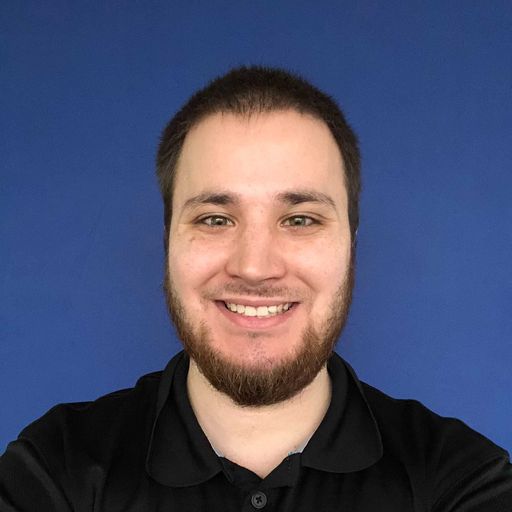
Devin Clark
Principal Software Engineer, Oracle
You Might Also Like